Estimated reading time: 6 minutes
Table of contents
Functional programming is a technique that deals with functions. Some prominent functional programming languages are Haskell, Scala, Erlang, Clojure, etc.
Function
The function is a piece of code that takes an input and gives back an output. Object Oriented Programming also has a concept of function. Object Oriented Programming function is a piece of code which is having some mechanism like :
- Deleting a file
- Updating a database
- Compute anything
- Compute nothing
- Return anything
- Return nothing
Functional Programming functions are different from Object Oriented functions. Functional programming functions are known as Mathematical functions.
Mathematical Function
It is a relation from a set of inputs to a set of possible outputs, where each input is related to exactly one output. Consider the example below :
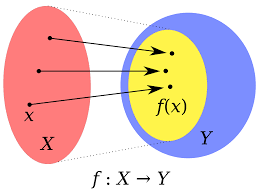
input : [ 1 , 2 , 3 , 4 , 5 ]
output : [ 2 , 4 , 6 , 8 , 10 ]
Here, the element in the output doubles the element in the input. So we can write this function as :
f ( x ) = 2 x ;
The above mathematical function doubles the input. Such a type of function is a Pure Function. Pure Function always returns the same output when given the same input with no changes and no side effects.
Functional approach
A basic rule of functional programming language is the input should not be changed.
x = [ 1 , 2 , 3 , 4 , 5 ]
y = f ( x )
x = ? Here, x should be the same, it should not change that is the rule.
We are aware of data structures like arrays, list, map, etc which are mutable. We can modify them at any point in time in our code base as they are mutable. Consider a case where we are updating our input, check the example :
[ 1 , 2 , 3 , 4 , 5 ] -> [ 2 , 4 , 6 , 8 , 10 ]
Now while executing f ( x ) = 2 x, if we update the input array to [ 1 , 2 , 3 , 7 , 5 ] the result will be changed to: [ 1 , 2 , 3 , 4 , 5 ] -> [ 2 , 4 , 6 , 14 , 10 ]
Here we got a totally different output. And we have lost all the gains which we thought we got after using f ( x ). So a solution is to make the data structures immutable.
Overcoming Immutable Structure
We will ask our data structure to give a copy of the array with a modification ( i.e ) update the second last element to 7, so we will have a new input array [ 1 , 2, 3 , 7 , 5 ] . Now we got a proper input-output mapping
[ 1 , 2 , 3 , 7 , 5 ] -> [ 2 , 4 , 6 , 14 , 10 ]
But now we landed on a new problem of multiple copies. What if we have an array of millions of elements and we have to change 5 elements in the middle of that array? Should we change the first element and make a copy of million elements and change the second element and make other copies of million elements and so on?
No, some thinkers came up with an approach to store the elements in the form of a tree and it is known as persistent data structures. Consider the below example :
Input: 1 , 2 , 3 , 4 , 5 , 6 , 7 , 8 , 9 , 10 , 11 , 12 , 13 , 14
Branching factor
Suppose we are branching our input to a factor of three .
Input : [ 1 , 2 , 3 ] [ 4 , 5 , 6 ] [ 7 , 8 , 9 ] [ 10 , 11 , 12 ] [ 13 , 14 ]
Now if we want to update “10” to “15”, we will just make a copy of [ 10 , 11 , 12 ] and will keep other elements as it is and make use of them. The new array and old array share most of the data.
Updated input : 1 , 2 , 3 , 4 , 5 , 6 , 7 , 8 , 9 , 15 , 11 , 12 , 13 , 14
Here we just kept a factor of 3. But in real life, people keep a branching factor of almost 32. We got f ( x ), immutability, and Solution for copies and thus we achieved a functional programming approach and now we can work on actual data in the functional world.
Benefits of Functional Programming
- Higher level functions: A Function that can take other functions as its argument OR return a function as its result. Ex: Suppose you have to arrange a house
arrangeHouse ( bedroom, hall, kitchen )
Arranging a house means arranging the bedroom, hall, and kitchen separately, they are independent pieces and we should arrange them separately.
- Lazy Evaluation: It is a strategy that delays the evaluation of an expression until we don’t need its value. In the above example, we are passing the bedroom, hall, and kitchen to arrange the house but we won’t be arranging everything at once. We will divide our work thus when we want to arrange the bedroom we will use the bedroom’s functionality until the kitchen and hall are in an ideal state [ Load the functionality when required ]
- Recursive Function-Calls: Functional programming is pure with no side effects. Suppose we are using an iterative approach to iterate over a list using a counter. There is a high chance that we can update the counter and all the advantages of functional programming are lost hence instead of an iterative approach we will make use of recursion as no state is needed to recurse and we can implement it more effectively.
- Modular: Separating out independent parts of the program with minimum dependency between them.
Is functional Programming required for a project?
It depends on what kind of project you are working on. Suppose you are working mostly on Machine Learning, Game AIs, and mathematical computations where mathematics is of most importance then “Yes” is the answer.
But if we consider Real-world enterprise software like Vehicle Registration, Life insurance, E-Commerce, banking or payments software, etc then it is not that necessary to use functional programming because such software demands a complex set of requirements and abstractions. And as software developers, we have to fully satisfy the complex requirements demanded by the client.
Working as Object Oriented Programmers we can adopt the core constraints that drive the benefits of the functional paradigm. We can create a bridge between our object-oriented world and the functional world so that we can switch between the functional hat and object-oriented hat whenever needed.
Is Java purely functional?
Java is not purely functional. It is mainly an Object Oriented Programming language that allows us to adopt some functional concepts if needed.