Identifiers are names that are given to java elements . Using identifiers we can identify an element . An identifier can be a java variable name , method name , interface name , class name etc .
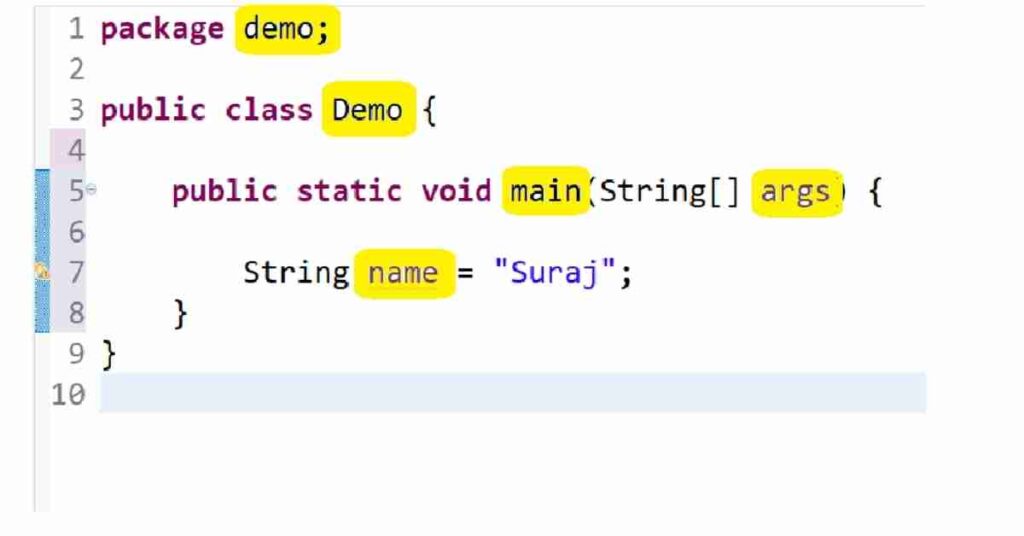
Rules for java identifiers
1 ) Characters that are allowed as java identifiers are
Alphabets : [ A – Z ] [ a – z ]
Digits : [ 0 – 9 ]
Special Charachters : [ $ or _ ]
If we are using any other character from the above-mentioned characters , we will get a Compile time exception .
package demo;
public class Demo {
public static void main(String[] args) {
String n@me = "Suraj";
}
}
Output : Exception in thread "main" java.lang.Error: Unresolved compilation problems: Syntax error on token "@", ; expected me cannot be resolved to a variable
2 ) Java identifiers are case-sensitive . We can differentiate an identifier by changing the case .
package demo;
public class Demo {
public static void main(String[] args) {
String name = "Suraj";
String Name = "Surya";
System.out.println(name + "-" + Name);
}
}
Output: Suraj - Surya
3 ) Java identifier cannot start with a digit . If we try to do that we will get a Compile time exception .
package demo;
public class Demo {
public static void main(String[] args) {
String 8name = "Suraj";
}
}
Output : Exception in thread "main" java.lang.Error: Unresolved compilation problem: Syntax error on token "8", delete this token
4 ) We cannot use reserved keywords as identifiers else we will get a Compile time exception .
package demo;
public class Demo {
public static void main(String[] args) {
String for = "Suraj";
}
}
Output: Exception in thread "main" java.lang.Error: Unresolved compilation problems: String cannot be resolved to a variable
Syntax error on token "for", delete this token
5 ) Identifier cannot have a space in the middle of words . If we do that we will get a compilation exception .
package demo;
public class Demo {
public static void main(String[] args) {
String my name = "Suraj";
}
}
Output: Exception in thread "main" java.lang.Error: Unresolved compilation problems: Syntax error, insert ";" to complete BlockStatements name cannot be resolved to a variable
If we want to connect multiple words to form an identifier then we can use
_ ( underscore ) to connect them .
package demo;
public class Demo {
public static void main(String[] args) {
String my_name = "Suraj";
System.out.println(my_name);
}
}
Output: Suraj
6 ) All predefined java interface names and class names can be used as identifiers .
package demo;
public class Demo {
public static void main(String[] args) {
String String = "Suraj";
System.out.println(String);
}
}
Output: Suraj
Even though it is possible to use existing class and interface names as identifiers we should never do this because this is against java’s good programming practices .
7 ) There is no max length limit for java identifiers but it is suggested to keep the identifiers of smaller length .
In this article , we have covered about java identifiers and the rules for java
identifiers . I hope you found this article interesting and valuable . Please share this article with your friends and help me grow . If you are having any concerns or questions about this article please comment below . If you want to get in touch with me please visit the Contact Me page and send me an email .
1 thought on “Java Identifiers and the rules”